Example - Beginner 5x5 modified¶
(inspired by stronglifts)
This notebook shows features of streprogen, the Python strength program generator.
Contributions to the code are welcome. :)
[1]:
!pip install streprogen matplotlib --quiet
Imports¶
[2]:
from streprogen import Program, reps_to_intensity, progression_diffeq
import matplotlib.pyplot as plt
import functools
Choose level of non-linearity¶
By default, streprogen
introduces a little non-linearity in the general strength progression, see below.
[3]:
plt.figure(figsize=(8, 4))
plt.title("Relationship between weeks and general strength progression")
duration = 12
weeks = list(range(1, duration + 1))
for k in range(0, 4):
progression = functools.partial(progression_diffeq, k=k)
plt.plot(weeks, progression(weeks, start_weight=100, final_weight=120,
start_week=1, final_week=12), '-o', label="k=" + str(k))
plt.xlabel("Weeks"); plt.ylabel("Weight")
plt.grid(); plt.legend(); plt.tight_layout()
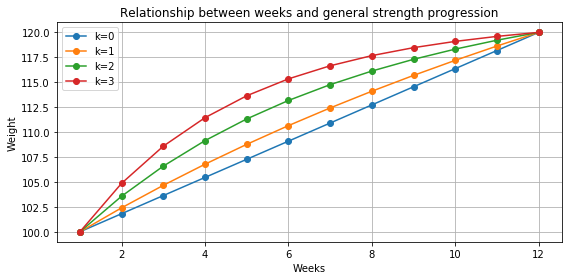
You can choose the non-linearity used below. Setting k=0
removes all non-linearity.
[4]:
# Setting k=0 means linear progression.
# The default value is slightly higher than 0.
k = 1
progression = functools.partial(progression_diffeq, k=k)
Program setup¶
Below is the code creating the program.
- We modify the 5x5 scheme by allowing reps to be between 4 and 6.
- The default settings will add some some periodicity to the number of reps and the intensity over the weeks.
[5]:
# Increase in percentage per week. If set to 1.5, it takes you from 100kg to 103kg in 2 weeks.
# Here day A and B are cycled with 3 workouts per week, so we set it to a reasonably low value.
percent_inc_per_week = 1.4
program = Program(
# The name of the training program
name='Beginner5x5modified',
# The duration of the training program in weeks.
# Here day A and B are cycled with 3 workouts per week, so the duration would be ~8 weeks
duration=12,
# The baseline number of repetitions per dynamic exercise.
reps_per_exercise=22,
min_reps=3,
max_reps=7,
percent_inc_per_week=percent_inc_per_week,
# The baseline intensity value (approx 82 %)
intensity=reps_to_intensity(5),
# Units for the weights, typically 'kg', 'lbs' or '' (empty)
units='kg',
# What the weights are rounded to.
round_to=2.5,
# Override the default progression function with out own
progression_func=progression
)
# --------------------------------------------------
# ---- INPUT YOUR OWN 1RMs AS START WEIGHTS BELOW --
# ---- Carefully assess the program, then go back --
# ---- and adjust further if necessary. --
# --------------------------------------------------
with program.Day("A"):
program.DynamicExercise(name="Squat", start_weight=80)
program.DynamicExercise(name="Bench Press", start_weight=60)
program.DynamicExercise(name="Barbell Row", start_weight=50)
with program.Day("B"):
program.DynamicExercise(name="Squat", start_weight=80)
program.DynamicExercise(name="Overhead Press", start_weight=40)
# Notice the additional `reps=5` here, constraining this exercise to a single set.
# This overrides the `reps_per_exercise=25` parameter in the program for this exercise.
program.DynamicExercise(name="Deadlift", start_weight=80, reps=5)
Render the program¶
[6]:
# Do the computations and render a program. Might take a few seconds.
program.render()
Print and save the program¶
[7]:
print(program)
----------------------------------------------------------------
Program: Beginner5x5modified
Program parameters
duration: 12
reps_per_exercise: 22
intensity: 84.3
units: kg
----------------------------------------------------------------
Exercise information
A
Squat 80kg -> 93.4kg
reps: [3, 7] weekly inc.: 1.4%
Bench Press 60kg -> 70.1kg
reps: [3, 7] weekly inc.: 1.4%
Barbell Row 50kg -> 58.4kg
reps: [3, 7] weekly inc.: 1.4%
B
Squat 80kg -> 93.4kg
reps: [3, 7] weekly inc.: 1.4%
Overhead Press 40kg -> 46.7kg
reps: [3, 7] weekly inc.: 1.4%
Deadlift 80kg -> 93.4kg
reps: [3, 7] weekly inc.: 1.4%
----------------------------------------------------------------
Program
Week 1
A
Squat 7 x 62.5kg 7 x 62.5kg 6 x 65kg 6 x 65kg
Bench Press 7 x 47.5kg 7 x 47.5kg 6 x 50kg 6 x 50kg
Barbell Row 7 x 40kg 7 x 40kg 6 x 40kg 6 x 40kg
B
Squat 7 x 62.5kg 7 x 62.5kg 6 x 65kg 6 x 65kg
Overhead Press 7 x 32.5kg 7 x 32.5kg 6 x 32.5kg 6 x 32.5kg
Deadlift 6 x 65kg
Week 2
A
Squat 7 x 65kg 6 x 67.5kg 6 x 67.5kg 6 x 67.5kg
Bench Press 7 x 47.5kg 6 x 50kg 6 x 50kg 6 x 50kg
Barbell Row 7 x 40kg 6 x 42.5kg 6 x 42.5kg 6 x 42.5kg
B
Squat 7 x 65kg 6 x 67.5kg 6 x 67.5kg 6 x 67.5kg
Overhead Press 7 x 32.5kg 6 x 32.5kg 6 x 32.5kg 6 x 32.5kg
Deadlift 6 x 67.5kg
Week 3
A
Squat 6 x 67.5kg 6 x 67.5kg 6 x 67.5kg 6 x 67.5kg
Bench Press 6 x 50kg 6 x 50kg 6 x 50kg 6 x 50kg
Barbell Row 6 x 42.5kg 6 x 42.5kg 6 x 42.5kg 6 x 42.5kg
B
Squat 6 x 67.5kg 6 x 67.5kg 6 x 67.5kg 6 x 67.5kg
Overhead Press 6 x 35kg 6 x 35kg 6 x 35kg 6 x 35kg
Deadlift 6 x 67.5kg
Week 4
A
Squat 6 x 67.5kg 6 x 67.5kg 6 x 67.5kg 5 x 72.5kg
Bench Press 6 x 52.5kg 6 x 52.5kg 6 x 52.5kg 5 x 52.5kg
Barbell Row 6 x 42.5kg 6 x 42.5kg 6 x 42.5kg 5 x 45kg
B
Squat 6 x 67.5kg 6 x 67.5kg 6 x 67.5kg 5 x 72.5kg
Overhead Press 6 x 35kg 6 x 35kg 6 x 35kg 5 x 35kg
Deadlift 6 x 67.5kg
Week 5
A
Squat 6 x 70kg 6 x 70kg 5 x 72.5kg 5 x 72.5kg
Bench Press 6 x 52.5kg 6 x 52.5kg 5 x 55kg 5 x 55kg
Barbell Row 6 x 42.5kg 6 x 42.5kg 5 x 45kg 5 x 45kg
B
Squat 6 x 70kg 6 x 70kg 5 x 72.5kg 5 x 72.5kg
Overhead Press 6 x 35kg 6 x 35kg 5 x 35kg 5 x 35kg
Deadlift 5 x 72.5kg
Week 6
A
Squat 6 x 70kg 5 x 72.5kg 5 x 72.5kg 5 x 72.5kg
Bench Press 6 x 52.5kg 5 x 55kg 5 x 55kg 5 x 55kg
Barbell Row 6 x 45kg 5 x 45kg 5 x 45kg 5 x 45kg
B
Squat 6 x 70kg 5 x 72.5kg 5 x 72.5kg 5 x 72.5kg
Overhead Press 6 x 35kg 5 x 37.5kg 5 x 37.5kg 5 x 37.5kg
Deadlift 5 x 72.5kg
Week 7
A
Squat 5 x 75kg 5 x 75kg 5 x 75kg 4 x 77.5kg 3 x 80kg
Bench Press 5 x 55kg 5 x 55kg 5 x 55kg 4 x 57.5kg 3 x 60kg
Barbell Row 5 x 47.5kg 5 x 47.5kg 5 x 47.5kg 4 x 47.5kg 3 x 50kg
B
Squat 5 x 75kg 5 x 75kg 5 x 75kg 4 x 77.5kg 3 x 80kg
Overhead Press 5 x 37.5kg 5 x 37.5kg 5 x 37.5kg 4 x 37.5kg 3 x 40kg
Deadlift 5 x 75kg
Week 8
A
Squat 5 x 75kg 5 x 75kg 4 x 77.5kg 4 x 77.5kg 3 x 80kg
Bench Press 5 x 57.5kg 5 x 57.5kg 4 x 57.5kg 4 x 57.5kg 3 x 60kg
Barbell Row 5 x 47.5kg 5 x 47.5kg 4 x 50kg 4 x 50kg 3 x 50kg
B
Squat 5 x 75kg 5 x 75kg 4 x 77.5kg 4 x 77.5kg 3 x 80kg
Overhead Press 5 x 37.5kg 5 x 37.5kg 4 x 40kg 4 x 40kg 3 x 40kg
Deadlift 5 x 75kg
Week 9
A
Squat 5 x 77.5kg 5 x 77.5kg 4 x 80kg 3 x 82.5kg 3 x 82.5kg
Bench Press 5 x 57.5kg 5 x 57.5kg 4 x 60kg 3 x 62.5kg 3 x 62.5kg
Barbell Row 5 x 47.5kg 5 x 47.5kg 4 x 50kg 3 x 52.5kg 3 x 52.5kg
B
Squat 5 x 77.5kg 5 x 77.5kg 4 x 80kg 3 x 82.5kg 3 x 82.5kg
Overhead Press 5 x 37.5kg 5 x 37.5kg 4 x 40kg 3 x 40kg 3 x 40kg
Deadlift 4 x 80kg
Week 10
A
Squat 5 x 77.5kg 4 x 80kg 4 x 80kg 3 x 82.5kg 3 x 82.5kg
Bench Press 5 x 57.5kg 4 x 60kg 4 x 60kg 3 x 62.5kg 3 x 62.5kg
Barbell Row 5 x 47.5kg 4 x 50kg 4 x 50kg 3 x 52.5kg 3 x 52.5kg
B
Squat 5 x 77.5kg 4 x 80kg 4 x 80kg 3 x 82.5kg 3 x 82.5kg
Overhead Press 5 x 37.5kg 4 x 40kg 4 x 40kg 3 x 42.5kg 3 x 42.5kg
Deadlift 4 x 80kg
Week 11
A
Squat 5 x 77.5kg 4 x 80kg 3 x 85kg 3 x 85kg 3 x 85kg
Bench Press 5 x 57.5kg 4 x 60kg 3 x 62.5kg 3 x 62.5kg 3 x 62.5kg
Barbell Row 5 x 47.5kg 4 x 50kg 3 x 52.5kg 3 x 52.5kg 3 x 52.5kg
B
Squat 5 x 77.5kg 4 x 80kg 3 x 85kg 3 x 85kg 3 x 85kg
Overhead Press 5 x 40kg 4 x 40kg 3 x 42.5kg 3 x 42.5kg 3 x 42.5kg
Deadlift 4 x 80kg
Week 12
A
Squat 4 x 82.5kg 4 x 82.5kg 4 x 82.5kg 3 x 85kg 3 x 85kg
Bench Press 4 x 62.5kg 4 x 62.5kg 4 x 62.5kg 3 x 62.5kg 3 x 62.5kg
Barbell Row 4 x 50kg 4 x 50kg 4 x 50kg 3 x 52.5kg 3 x 52.5kg
B
Squat 4 x 82.5kg 4 x 82.5kg 4 x 82.5kg 3 x 85kg 3 x 85kg
Overhead Press 4 x 40kg 4 x 40kg 4 x 40kg 3 x 42.5kg 3 x 42.5kg
Deadlift 4 x 82.5kg
----------------------------------------------------------------
Export the program as .html
or .tex
, then to .pdf
¶
A .html
file can be printed directly from your browser, or printed to a .pdf
from your browser.
[8]:
# Save the program as a HTML file
with open('Beginner5x5modified.html', 'w', encoding='utf-8') as file:
# Control table width (number of sets) by passing the 'table_width' argument
file.write(program.to_html(table_width=8))
[ ]:
# Save the program as a TEX file
with open('Beginner5x5modified.tex', 'w', encoding='utf-8') as file:
# Control table width (number of sets) by passing the 'table_width' argument
file.write(program.to_tex(table_width=8))
Use a .tex
to generate .pdf
if you have LaTeX installed, or use:
- latexbase.com from your browser.
[ ]:
# If you have LaTeX installed on your system, you can render a program to .tex
# Alternatively, you can paste the LaTeX into: https://latexbase.com/
print(program.to_tex(table_width=8))